Example to builld AlertDialog with EditText and ImageView, build with AlertDialog.Builder.
Create layout/dialog_layout.xml, to define the layout of the dialog.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="This is custom layout in custom dialog"/>
<EditText
android:id="@+id/dialogEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
MainActivity.java
package com.blogspot.android_er.androidcustomalertdialog;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
Button btnOpenDialog;
TextView textInfo;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnOpenDialog = (Button)findViewById(R.id.opendialog);
textInfo = (TextView)findViewById(R.id.info);
btnOpenDialog.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
openDialog();
}
});
}
private void openDialog(){
LayoutInflater inflater = LayoutInflater.from(MainActivity.this);
View subView = inflater.inflate(R.layout.dialog_layout, null);
final EditText subEditText = (EditText)subView.findViewById(R.id.dialogEditText);
final ImageView subImageView = (ImageView)subView.findViewById(R.id.image);
Drawable drawable = getResources().getDrawable(R.mipmap.ic_launcher);
subImageView.setImageDrawable(drawable);
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("AlertDialog");
builder.setMessage("AlertDialog Message");
builder.setView(subView);
AlertDialog alertDialog = builder.create();
builder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
textInfo.setText(subEditText.getText().toString());
}
});
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this, "Cancel", Toast.LENGTH_LONG).show();
}
});
builder.show();
}
}
layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:autoLink="web"
android:text="http://android-er.blogspot.com/"
android:textStyle="bold" />
<Button
android:id="@+id/opendialog"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Open Dialog"/>
<TextView
android:id="@+id/info"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="20dp"
android:textStyle="bold"/>
</LinearLayout>
Custom AlertDialog with EditText and ImageView, build with AlertDialog.Builder
Reviewed by Pendik
on
05.58
Rating:
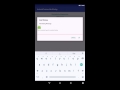
Tidak ada komentar: