A simple Android example to read info of RFID tag (key and card in this demo) using NFC.
Edit src/main/AndroidManifest.xml to add <intent-filter> with action of "android.nfc.action.TAG_DISCOVERED" and category of "android.intent.category.DEFAULT", such that the app will be started when RFID tag place near NFC; if no other apps registered.
And add <uses-permission> of "android.permission.NFC", and <uses-feature> of "android.hardware.nfc"
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.blogspot.android_er.androidnfctagdiscovered" >
<uses-permission android:name="android.permission.NFC"/>
<uses-feature android:name="android.hardware.nfc"
android:required="true"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme" >
<activity android:name=".MainActivity" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<intent-filter>
<action android:name="android.nfc.action.TAG_DISCOVERED"/>
<category android:name="android.intent.category.DEFAULT"/>
</intent-filter>
</activity>
</application>
</manifest>
In MainActivity.java:
In onCreate():
Create NfcAdapter object by calling NfcAdapter.getDefaultAdapter(this). And check if NFC supported and enabled.
Override onResume():
Check if intent is ACTION_TAG_DISCOVERED. if yes, we can obtain a Tag object from the intent, by calling intent.getParcelableExtra(NfcAdapter.EXTRA_TAG).
package com.blogspot.android_er.androidnfctagdiscovered;
import android.content.Intent;
import android.nfc.NfcAdapter;
import android.nfc.Tag;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
private NfcAdapter nfcAdapter;
TextView textViewInfo;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
textViewInfo = (TextView)findViewById(R.id.info);
nfcAdapter = NfcAdapter.getDefaultAdapter(this);
if(nfcAdapter == null){
Toast.makeText(this,
"NFC NOT supported on this devices!",
Toast.LENGTH_LONG).show();
finish();
}else if(!nfcAdapter.isEnabled()){
Toast.makeText(this,
"NFC NOT Enabled!",
Toast.LENGTH_LONG).show();
finish();
}
}
@Override
protected void onResume() {
super.onResume();
Intent intent = getIntent();
String action = intent.getAction();
if (NfcAdapter.ACTION_TAG_DISCOVERED.equals(action)) {
Toast.makeText(this,
"onResume() - ACTION_TAG_DISCOVERED",
Toast.LENGTH_SHORT).show();
Tag tag = intent.getParcelableExtra(NfcAdapter.EXTRA_TAG);
if(tag == null){
textViewInfo.setText("tag == null");
}else{
String tagInfo = tag.toString() + "\n";
tagInfo += "\nTag Id: \n";
byte[] tagId = tag.getId();
tagInfo += "length = " + tagId.length +"\n";
for(int i=0; i<tagId.length; i++){
tagInfo += Integer.toHexString(tagId[i] & 0xFF) + " ";
}
tagInfo += "\n";
String[] techList = tag.getTechList();
tagInfo += "\nTech List\n";
tagInfo += "length = " + techList.length +"\n";
for(int i=0; i<techList.length; i++){
tagInfo += techList[i] + "\n ";
}
textViewInfo.setText(tagInfo);
}
}else{
Toast.makeText(this,
"onResume() : " + action,
Toast.LENGTH_SHORT).show();
}
}
}
layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:layout_gravity="center_horizontal"
android:autoLink="web"
android:text="http://android-er.blogspot.com/"
android:textStyle="bold"/>
<TextView
android:id="@+id/info"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
![]() |
Tag info of RFID card |
![]() |
Tag info of RFID key |
Next:
- Android NFC read MifareClassic RFID tag, with android.nfc.action.TECH_DISCOVERED
- Android NFC: readBlock() for MifareClassic, to dump data in RFID tag
Android NFC example, to read tag info of RFID key and card
Reviewed by Pendik
on
06.02
Rating:
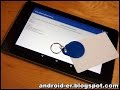
Thanks for sharing this blog, this blog is very helpful information for every one.
BalasHapusRFID key cards
RFID key cards hotel
Custom hotel key cards